MonopoleGenerator¶
The Monopole-Generator project provides two modules for IceCube
simulations: The I3MonopoleGenerator
generates randomly distributed
magnetic-monopole particles. The I3MonopolePropagator
then
propagates those monopole particles. Along their trajectories, the
propagator decreases their energy to account for ionization effects and
creates secondary particles from interactions of the monopole particles
with the surrounding medium.
Usage¶
tray.AddService("I3GSLRandomServiceFactory", "random")
tray.AddModule("I3InfiniteSource", "infinite", Stream = icetray.I3Frame.DAQ)
tray.AddModule("I3MonopoleGenerator", "generator")(
("NEvents", 1e5),
("TreeName", "I3MCTree"),
("InfoName", "MPInfoDict"),
("Disk_dist", 1000 * icetray.I3Units.m),
("Disk_rad", 850 * icetray.I3Units.m),
("BetaRange", [0.4, 0.995]),
("powerLawIndex", 5.),
("Mass", 1e11 * icetray.I3Units.GeV)
)
tray.AddModule("I3MonopolePropagator", "propagator")(
("InputTreeName", "I3MCTree"),
("OutputTreeName", "I3MCTree"),
("InfoName", "MPInfoDict"),
("MinLength", 1. * icetray.I3Units.m),
("MaxLength", 10. * icetray.I3Units.m)
)
For a working example, see resources/examples/PlotGeneratingDistributions.py.
Monopole-Generator Parameters¶
NEvents (Default: 0) Number of events to be generated for the simulation.
TreeName (Default: “I3MCTree”) Name of the
I3MCTree
to write the generated monopole particle to.InfoName (Default: “MPInfoDict”) Name of the monopole info dictionary. The generator writes generation parameters into this dictionary.
Disk_dist (Default:
1000 * I3Units::m
) Distance of the generation disk from the center of IceCube.Disk_rad (Default:
800 * I3Units::m
) Radius of the generation disk.BetaRange (Default:
[NaN, NaN]
) Velocity range of the monopole particle as array of lower and upper boundary. If a fixed velocity is desired, set lower and upper boundary to the same velocity. Express the velocities as ratio of the speed of light, c. For example:[0.1, 0.4]
.PowerLawIndex (Default:
NaN
) If a power-law index is given, the velocities of the simulated monopole particles will follow a power-law distribution with the given index. This can be helpful ti gain statistics for low velocities. If no power-law index is given, the velocities will be uniformly distributed (default).Mass (Default:
NaN
) Mass of the monopole particle. For slow monopoles, this parameter is optional. For fast monopoles, this parameter is needed to calculate energy losses along the trajectory. Example:1e7 * I3Units::GeV
.Length (Default:
2 * Disk_dist
) Length of the monopole track. Can beNaN
or any length. The default value is calculated to twice the disk distance. Set to-1
to indicate that the default value,2 * Disk_dist
, should be used.ZenithRange (Default:
[0.0 * I3Units::deg, 180.0 * I3Units::deg]
) List of lower and upper zenith bound. Use this parameter to restrict the direction of the monopole. Example:[0.0 * I3Units::deg, 180.0 * I3Units::deg]
AzimuthRange (Default:
[0.0 * I3Units::deg, 360.0 * I3Units::deg]
) List of lower and upper azimuth bound. Use this parameter to restrict the direction of the monopole. Example:[0.0 * I3Units::deg, 360.0 * I3Units::deg]
Rad_on_disk (Default:
NaN
) Set the radius coordinate of the starting position on the generation disk. Randomized ifNaN
. Example:5. * I3Units::m
Azi_on_disk (Default:
NaN
) Set the azimuth coordinate of the starting position on the generation disk. Randomized ifNaN
. Example:45. * I3Units::deg
ShiftCenter (Default:
[0., 0., 0.]
) Shifts the monopole. This is useful to explore different geometries. To shift according to the center of DeepCore (IC86-I SLOP trigger only acts on DC), configure withShiftCenter = ([46.0 * icetray.I3Units.m, -34.5 * icetray.I3Units.m, -330.0 * icetray.I3Units.m])
.StartTime (Default:
0. * I3Units::s
) The time, measured from the beginning of the event, the monopole particle should be started. Example:0. * I3Units::s
Monopole-Propagator Parameters¶
InputTreeName (Default: “I3MCTree”) Name of the
I3MCTree
containing the monopole generated by the monopole generator.OutputTreeName (Default: “I3MCTree”) Name of the
I3MCTree
to write the monopole into after propagation.InfoName (Default: “MPInfoDict”) Name of the monopole info dictionary, containing all necessary information about the generation parameters.
BetaThreshold (Default: 0.09) Threshold that determines whether the monopole propagator handles the monopole particle as slow (non-relativistic) or fast (relativistic) particle. This determines the interaction types the propagator will consider.
Parameters for non-relativistic (“slow”) monopole particles:
MeanFreePath (Default:
NaN
) Mean free path (lambda) between catalyzed proton decays. Example:1 * I3Units::m
ScaleEnergy (Default:
false
) Whether to set the mean free path (lambda) to 1 meter and scale up the energy by 1/lambda. The overall light output stays comparable, while the number of secondary particles in theI3MCTree
is reduced. This saves computing resources especially for short mean free paths.UseCorrectDecay (Default:
false
) Whether to simulate back-to-back positrons (460 MeV) and neutral pions (480 MeV) instead of just one positron. The overall light output is similar, but correct decay has twice as much secondary particles in theI3MCTree
. This option cannot be used together withScaleEnergy
orEnergyScaleFactor
, since the energies are hard coded.EnergyScaleFactor (Default: 1.0) Scale down the cascade energy in order to test the influence of other decay channels.
Parameters for relativistic (“fast”) monopole particles:
CalculateEnergy (Default:
true
) If set totrue
, the energy loss of the monopole particles due to ionization effects is calculated during the propagation. If set tofalse
, the monopole-particle energy and velocity are not decreasing during propagation.MaxDistanceFromCenter (Default:
800 * I3Units::m
) How far beyond the detector to propagate the monopole. If the start of the monopole is further from the detector than this value, the propagator will IGNORE the parameter and propagate until it reaches the same distance away on far side of detector. Example:800 * I3Units::m
Profiling (Default:
false
) Iftrue
, adds a profile (typeI3VectorDouble
) of the monopole speed for each track segment to the frame.SpeedMin (Default:
0.09 * I3Constants::c
) The speed at which the propagator should stop propagating. The value should not be set below around 0.1c if one is usingCalculateEnergy
, since the ionization formula assumes it above this range. If you use a lower speed min, please make sure you turn offCalculateEnergy
and treat this as a track segmentor only. Example:0.09 * I3Constants::c
StepSize (Default:
NaN
) Length of monopole track segments. If set this will overrideMinLength
andMaxLength
. Otherwise,MinLength
andMaxLength
are used to set the lower and upper bounds on the track segment lengths. Example:1 * I3Units::m
MinLength (Default:
0.001 * I3Units::m
) AssumingStepSize
isNaN
, this represents the smallest segment the propagator will generate. Example:0.001 * I3Units::m
MaxLength (Default:
10 * I3Units::m
) AssumingStepSize
isNaN
, this represents the largest segment the propagator will generate. Example:10 * I3Units::m
Background Information¶
The simulation chain that determines the response of the IceCube detector to particles passing through the detector consists of four main components: Generators, propagators, light injectors, and the detector simulation.
This project provides a generator for magnetic-monopole particles and a propagator for these particles.
Generating Magnetic-Monopole Particles in Simulations¶
The monopole-generator module creates and initializes the basic data structures needed by the other components of the simulation chain. The monopole particles’ starting points are randomly placed on a disk (“generation plane”) with a starting direction perpendicular to the disk as shown in the figure below. The disk itself is randomly rotated around the detector center to simulate an isotropic flux. This module supports the simulation of relativistic (“fast”) and sub-relativistic (“slow”) monopole particles, independent of their interaction types.
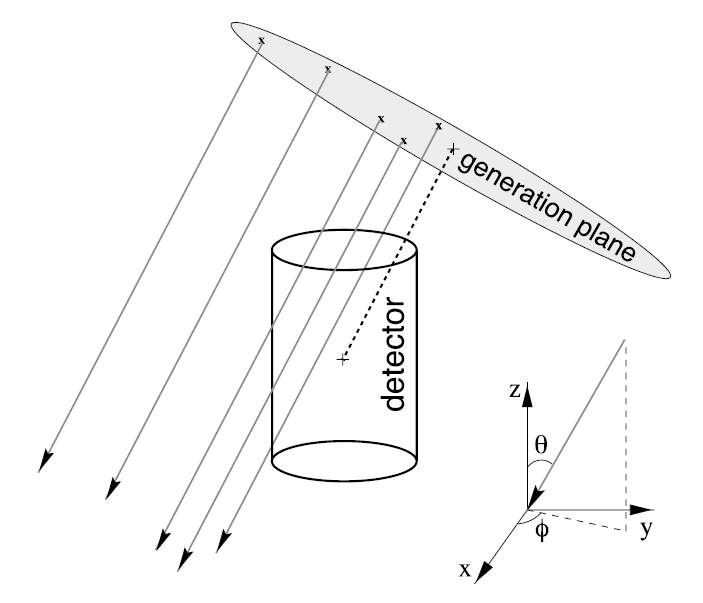
monopole generation disk¶
I3MonopoleGenerator
is the I3Module
to generate monopole events.
For each event, an I3MCTree
is created that contains a monopole as
its primary I3Particle
. An info dictionary containing important
simulation parameters is also written for each event and saved in the i3
file.
The velocity of the monopole (expressed in either BetaRange
or
Gamma
) is a mandatory parameter and no default is given. If
providing a range, the generator simulates a uniform velocity
distribution in the given range. If you define a power-law index
additionally, a power-law distribution is simulated and the
corresponding weights are written to the info dictionary.
For relativistic monopoles (faster than about 0.1c), a Mass
parameter is required as well, in order to calculate the energy loss
during the propagation.
The radius and distance of the generation plane have to be chosen depending on the detector size in order to obtain an isotropic flux. The default should be okay for the IC86 detector.
For directional studies, the zenith and azimuth of the monopole direction can be restricted to an interval. If the lower bound equals the upper bound the random generator will not be invoked and the monopole will be generated with a fixed direction. Furthermore, the starting position on the generation disk can be set to a fixed value.
Propagating Magnetic-Monopole Particles in Simulations¶
The monopole-propagator module moves the generated magnetic-monopole particles through the medium. It iteratively updates the monopole-particle position and simulates the interaction of the monopole particle with the medium.
Depending on the monopole-particle velocity stored in the info directory, the propagation module handles the following interaction types:
For non-relativistic (“slow”) monopole particles: Catalysis of proton decays
For relativistic (“fast”) monopole particles: Luminescence, indirect, and direct Cherenkov-light emission
For fast monopole particles, the propagator calculates the energy loss
of the particle per simulation step depending on the monopole-particle
mass and updates the monopole-particle velocity in each simulation step
accordingly. The module returns an MCTree
containing many particles
lined up in order to track the velocity changes.
For slow monopole particles, the propagator adds Poisson-distributed
numbers of cascades at uniformly distributed positions along the
monopole particles’ tracks. By default, for each catalyzed proton decay,
a positron (“eplus”) is produced, carrying the whole energy from the
proton. Although this is not correct physics-wise, the light output is
the same, as the direction of each cascade is randomized as well.
Nevertheless, there is the option to use the correct decay
(UseCorrectDecay
) into a 460-MeV positron and a 480-MeV neutral pion
at the cost of twice as much secondary particles in the MCTree
,
which have to be propagated thorough the detector. To save further
computing resources, the option ScaleEnergy
allows to reduce the
number of cascades (stipulates a mean free path of 1m) while increasing
their energy. This is helpful for short mean free paths.
Installation¶
Clone the source code of IceCube’s Combo framework:
$ export ICECUBE_COMBO_ROOT="~/combo"
$ export ICECUBE_COMBO_SRC="$ICECUBE_COMBO_ROOT/src"
$ export ICECUBE_COMBO_BUILD="$ICECUBE_COMBO_ROOT/build"
$ git clone git@github.com:IceCube-SPNO/IceTrayCombo.git $ICECUBE_COMBO_SRC
If there is a $ICECUBE_COMBO_SRC/monopole-generator
directory, the
monopole-generator modules are already provided by combo. Otherwise,
clone the source code of the monopole generator as well:
$ git clone git@github.com:fiedl/monopole-generator.git $ICECUBE_COMBO_SRC/monopole-generator
Then configure and compile the software:
$ cd $ICECUBE_COMBO_BUILD
$ cmake -D CMAKE_BUILD_TYPE=Debug -D SYSTEM_PACKAGES=true $ICECUBE_COMBO_SRC
$ ./env-shell.sh make -j 8
To check whether the installation was successful, run the example script:
$ cd $ICECUBE_COMBO_BUILD
$ ./env-shell.sh python $ICECUBE_COMBO_SRC/monopole-generator/resources/examples/PlotGeneratingDistributions.py
$ open *.png
An install script used for continuous integration tests, can be found here: fiedl/icecube-combo-install, fiedl/monopole-generator-install.
Running Tests¶
This project includes python tests and cpp tests.
# run the cpp tests
$ cd $ICECUBE_COMBO_BUILD
$ ./env-shell.sh bash -c "cd monopole-generator && make monopole-generator-test"
$ ./env-shell.sh bin/monopole-generator-test --all
# run the python tests
$ cd $ICECUBE_COMBO_BUILD
$ ./env-shell.sh python $ICECUBE_COMBO_SRC/monopole-generator/resources/test/test_monopole_generator.py
$ ./env-shell.sh python $ICECUBE_COMBO_SRC/monopole-generator/resources/test/test_monopole_propagator.py
Documentation¶
To inspect the interface of the monopole-generator project, use icetray-inspect
$ cd $ICECUBE_COMBO_BUILD
$ ./env-shell.sh icetray-inspect monopole-generator
To generate and inspect the documentation for classes and variables, run:
$ cd $ICECUBE_COMBO_BUILD
$ ./env-shell.sh make docs
$ open docs/index.html
In order to keep documentation synchronized, please sync this
README.md
file after editing to the doxygen
documentation using the
pandoc converter:
$ brew install pandoc
$ pandoc --from markdown --to rst README.md > resources/docs/index.rst
Contributing¶
If you do encounter a bug or you would like to make a suggestion, please create an issue on github here or here.
If you would like to contribute code or make other changes to this project, please create a pull request on github. Make sure that the tests succeed before committing.
Don’t hesitate to slack for any questions you might have.
Code Review¶
Resources¶
Papers and Publications¶
Pollmann, Searches for magnetic monopoles with IceCube, 2018
IceCube, Searches for relativistic magnetic monopoles in IceCube, 2016
IceCube, Search for non-relativistic magnetic monopoles with IceCube, 2014
Christy, A Search for Relativistic Magnetic Monopoles With the IceCube 22-String Detector, PhD thesis, 2011
Glüsenkamp, On the Detection of Subrelativistic Magnetic Monopoles with the IceCube Neutrino Observatory, PhD thesis, 2010
Callan, Monopole Catalysis of Baryon Decay, 1983
Rubakov, Adler-Bell-Jackiw Anomaly and Fermion Number Breaking in the Presence of a Magnetic Monopole, 1982
Dirac, Quantised Singularities in the Electromagnetic Field, 1931
IceCube Wiki¶
Magnetic-Monopole Theory, Posselt, 2011
Relativistic-Monopole Simulation, Posselt, 2011
Background Generation for Slow Monopoles, Glüsenkamp, 2010